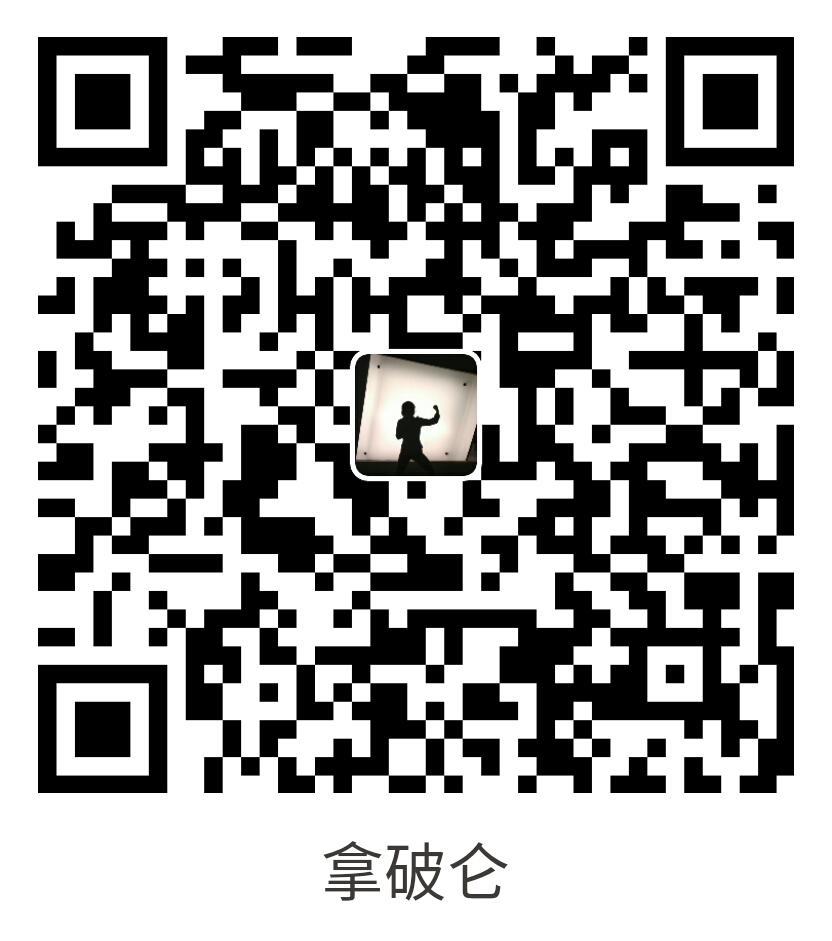
支付宝扫一扫付款
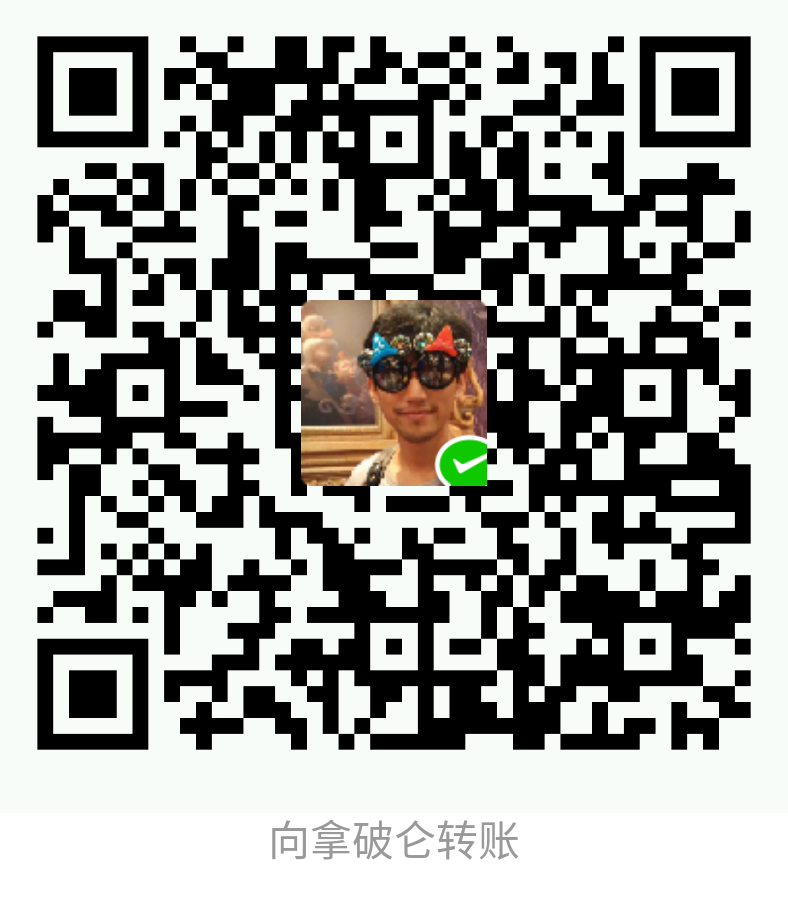
微信扫一扫付款
(微信为保护隐私,不显示你的昵称)
有时,你需要面对自定义格式(format)的翻译源。Translation组件足够灵活,可以支持它。只需创建一个加载器(loader,用于加载翻译文件),以及,一个可选的dumper(用于剥离出翻译内容)。
设想你有一个自定义的格式,里面的翻译信息(message)使用“单行的内容”来加以定义,用括号包住了键和message。翻译文件看上去像下面这样:
1 2 3 | (welcome)(accueil)
(goodbye)(au revoir)
(hello)(bonjour) |
要定义一个客制化loader,令其能够读取这些文件的内容,你必须创建一个全新的类,该类实现 LoaderInterface
接口。load()
方法将获取到文件名并把它解析成数组。然后,它会创建将被返回的目录:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 | use Symfony\Component\Translation\MessageCatalogue;
use Symfony\Component\Translation\Loader\LoaderInterface;
class MyFormatLoader implements LoaderInterface
{
public function load($resource, $locale, $domain = 'messages')
{
$messages = array();
$lines = file($resource);
foreach ($lines as $line) {
if (preg_match('/\(([^\)]+)\)\(([^\)]+)\)/', $line, $matches)) {
$messages[$matches[1]] = $matches[2];
}
}
$catalogue = new MessageCatalogue($locale);
$catalogue->add($messages, $domain);
return $catalogue;
}
} |
一旦创建,它就可以作为“其他类型”的loader来使用:
1 2 3 4 5 6 7 8 | use Symfony\Component\Translation\Translator;
$translator = new Translator('fr_FR');
$translator->addLoader('my_format', new MyFormatLoader());
$translator->addResource('my_format', __DIR__.'/translations/messages.txt', 'fr_FR');
var_dump($translator->trans('welcome')); |
它将输出 "accueil"。
针对你的文件格式来创建一个自定义的dumper也是可以的,这在使用提取命令时(extraction commands)很有用。这么做时,必须创建一个实现了 DumperInterface
接口的类。把剥离出的内容写入文件时,继承 FileDumper
类以便少写几行代码:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 | use Symfony\Component\Translation\MessageCatalogue;
use Symfony\Component\Translation\Dumper\FileDumper;
class MyFormatDumper extends FileDumper
{
public function formatCatalogue(MessageCatalogue $messages, $domain, array $options = array())
{
$output = '';
foreach ($messages->all($domain) as $source => $target) {
$output .= sprintf("(%s)(%s)\n", $source, $target);
}
return $output;
}
protected function getExtension()
{
return 'txt';
}
} |
formatCatalogue()
创建了output字符串,可以被FileDumper类的 dump()
方法用来创建文件。dumper可以像其他内置的dumper那样使用。在下例中,定义在YAML文件中的翻译信息将被按照指定格式被剥离成一个文件文件:
1 2 3 4 5 6 7 | use Symfony\Component\Translation\Loader\YamlFileLoader;
$loader = new YamlFileLoader();
$catalogue = $loader->load(__DIR__ . '/translations/messages.fr_FR.yml' , 'fr_FR');
$dumper = new MyFormatDumper();
$dumper->dump($catalogue, array('path' => __DIR__.'/dumps')); |
本文,包括例程代码在内,采用的是 Creative Commons BY-SA 3.0 创作共用授权。