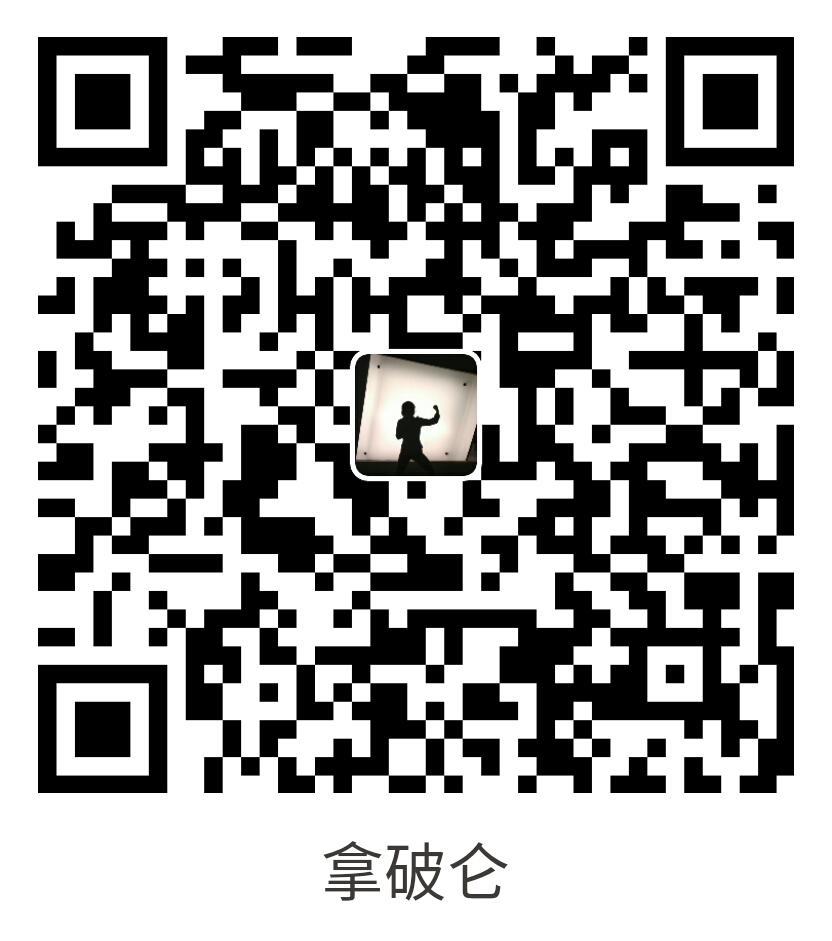
支付宝扫一扫付款
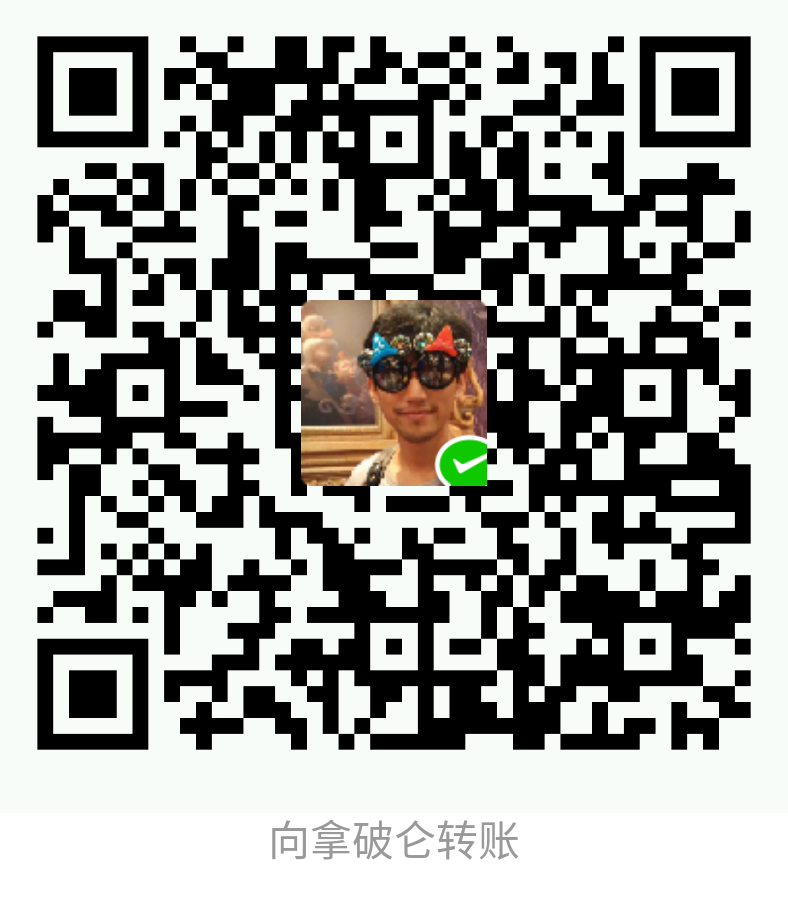
微信扫一扫付款
(微信为保护隐私,不显示你的昵称)
IniFileLoader
在解析文件内容时,用的是parse_ini_file
函数。因此,你只能设置(文件中配置信息的)参数(parameters)为字符串值。要设置参数为其他值类型(比如,boolean, integer, 等等),推荐使用其他加载器。
要加载配置文件,通常始于对资源的搜索,多数时候是对文件。这可以通过 FileLocator
来完成:
1 2 3 4 5 6 | use Symfony\Component\Config\FileLocator;
$configDirectories = array(__DIR__.'/app/config');
$locator = new FileLocator($configDirectories);
$yamlUserFiles = $locator->locate('users.yml', null, false); |
定位器(locator)接收一个“位置集合(数组)”,它在那里寻找文件。locate()
方法的第一个参数,是要寻找的文件名。第二个参数可以是当前路径,若提供了此参数,定位器将率先在此目录下寻找。第三个参数指明定位器是否应该返回它找到的第一个文件,或者,是否返回它所找到的包含全部匹配的一个数组。
对于每种资源 (YAML, XML, annotation, 等等) 必须定义一个加载器。每个加载器都要实现 LoaderInterface
接口,或者继承 FileLoader
抽象类,它允许递归式地导入资源:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 | use Symfony\Component\Config\Loader\FileLoader;
use Symfony\Component\Yaml\Yaml;
class YamlUserLoader extends FileLoader
{
public function load($resource, $type = null)
{
$configValues = Yaml::parse(file_get_contents($resource));
// ... handle the config values / 处理配置值
// maybe import some other resource: / 可能会导入其他资源
// $this->import('extra_users.yml');
}
public function supports($resource, $type = null)
{
return is_string($resource) && 'yml' === pathinfo(
$resource,
PATHINFO_EXTENSION
);
}
} |
LoaderResolver
的构造器第一个参数接收的是一个加载器集合。当一个资源(比如XML文件)要被加载时,它先遍历这个loader的数组,然后返回支持这个特定类型的那个加载器。
DelegatingLoader
利用的是 LoaderResolver
。当它被请求去加载一个资源时,它把此问题委托给了 LoaderResolver
。如果resolver(解析器)找到了一个合适的loader(加载器),那么这个loader会被要求去加载指定资源:
1 2 3 4 5 6 7 8 9 10 11 12 13 | use Symfony\Component\Config\Loader\LoaderResolver;
use Symfony\Component\Config\Loader\DelegatingLoader;
$loaderResolver = new LoaderResolver(array(new YamlUserLoader($locator)));
$delegatingLoader = new DelegatingLoader($loaderResolver);
$delegatingLoader->load(__DIR__.'/users.yml');
/*
The YamlUserLoader will be used to load this resource,
since it supports files with a "yml" extension
YamlUserLoader将被用于加载这个资源,
因为它支持“yml”扩展名的文件
*/ |
本文,包括例程代码在内,采用的是 Creative Commons BY-SA 3.0 创作共用授权。